System Design for Frontend Engineers: Building Scalable, Performant Applications
System design is often considered the domain of backend engineers, but as frontend applications grow in complexity, system design has become equally critical for frontend engineers. Designing scalable, maintainable, and performant frontends requires thoughtful consideration of architecture, data management, user experience, and integration with backend services. For frontend engineers aiming to elevate their technical skills, understanding system design principles is essential. Let’s explore the key concepts and strategies to approach system design from a frontend perspective.
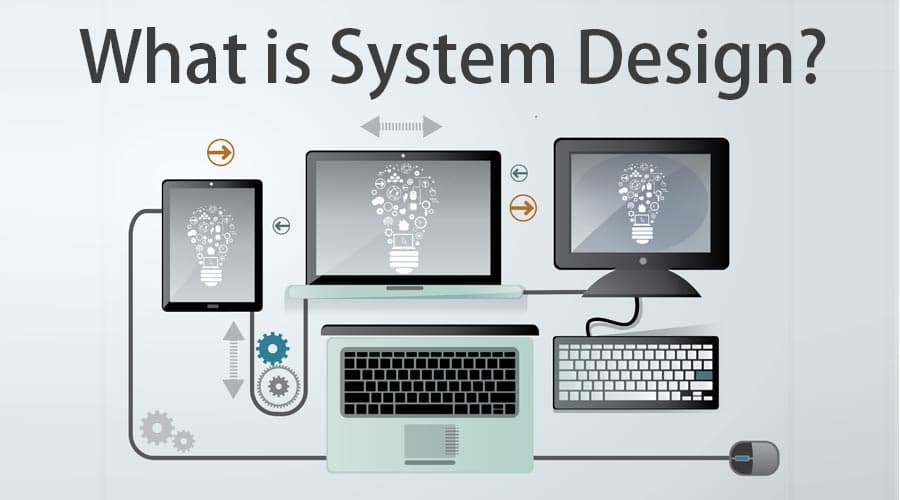
1. Component Architecture: Building Modular and Scalable UIs
At the heart of frontend system design is component architecture. Frontend applications are typically built using component-based frameworks like React, Vue, or Angular, where applications are composed of small, reusable components. A well-architected component system:
- Promotes Reusability: Thoughtful design ensures components can be reused across different parts of the application, reducing redundancy and development time.
- Enhances Maintainability: By creating small, self-contained components, teams can update or modify features with minimal impact on other parts of the application.
- Supports Scalability: Scalable component architecture supports growth, allowing additional features and pages to be added seamlessly.
When designing components, frontend engineers should also consider design patterns like atomic design, where components are broken down into atoms, molecules, and organisms for clear, hierarchical organization.
2. State Management: Efficiently Managing Data Across the Application
As frontend applications become more interactive, managing state across components and pages is essential. In system design, state management is the strategy to handle data effectively across the application. Key approaches include:
- Global State Management: For applications with a complex data flow, using tools like Redux, MobX, or the Context API helps manage data centrally and avoid “prop drilling” (passing data through many layers of components).
- Server State Management: For applications relying on real-time or frequently updated data, libraries like React Query or SWR can handle server state, caching, and data synchronization effectively, reducing the need for complex local state logic.
- Local Component State: Simpler components may only require local state. Managing state at the component level helps keep applications fast and avoids unnecessary re-renders.
Choosing the right state management strategy ensures that applications remain responsive, consistent, and scalable, especially as data requirements grow.
3. Performance Optimization: Delivering Fast, Smooth User Experiences
Performance is a critical factor in system design for frontend engineers. Optimizing frontend performance ensures users have fast, smooth interactions, improving satisfaction and retention. Key strategies include:
- Code Splitting: Dividing code into smaller bundles can significantly reduce load times. Tools like Webpack and strategies like lazy loading can optimize initial load and improve performance.
- Asset Optimization: Reducing the size of images, CSS, and JavaScript files is essential for fast-loading applications. Compression tools and content delivery networks (CDNs) help optimize these assets.
- Minimizing Re-Renders: Using techniques like React’s memoization (e.g.,
React.memo
oruseMemo
) helps reduce unnecessary re-renders, improving efficiency. - Efficient Data Fetching: Leveraging caching, pagination, and request bundling can prevent redundant network requests, ensuring efficient use of server resources.
Focusing on performance not only benefits user experience but also improves SEO and accessibility, making the application more discoverable and inclusive.
4. API Integration and Data Flow: Seamless Communication with Backend Services
Frontend applications need to communicate effectively with backend services. Designing an efficient data flow and robust API integration strategy ensures seamless interactions with backend servers. Considerations include:
- REST vs. GraphQL: Choosing between REST and GraphQL depends on data requirements. GraphQL allows clients to request only the data they need, which can improve efficiency for data-heavy applications.
- Error Handling and Resilience: Frontend engineers should design for resilience by handling API errors gracefully. This could mean displaying friendly error messages, retrying requests, or implementing fallback content.
- Data Security: Frontend systems should ensure secure data handling. Implementing token-based authentication, HTTPS, and securing user data are crucial in modern applications.
Thoughtful API design and data flow management are essential for creating responsive, reliable, and secure user experiences.
5. Accessibility and Usability: Creating Inclusive, User-Centered Designs
System design for frontend engineers must also account for accessibility and usability. Inclusive design ensures that the application can be used by a broad audience, including those with disabilities. Considerations include:
- Semantic HTML: Using HTML elements properly (e.g.,
<button>
,<header>
,<nav>
) ensures screen readers and assistive technologies interpret the content accurately. - Keyboard Navigation: Implementing proper keyboard navigation enables users to interact with the application without a mouse, improving usability for all.
- ARIA Roles: Accessible Rich Internet Applications (ARIA) roles and attributes provide additional context to assistive technologies, enhancing the experience for users with visual impairments.
By designing with accessibility in mind, frontend engineers can create inclusive applications that serve a wider audience, meeting legal standards and corporate values for inclusivity.
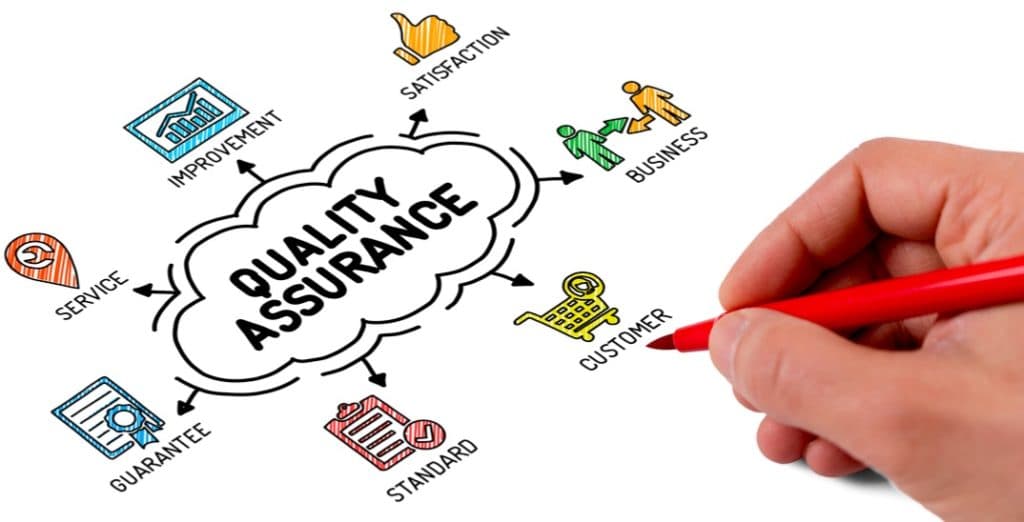
6. Continuous Testing and Quality Assurance: Ensuring Reliability and Stability
Testing is integral to frontend system design, ensuring that applications are reliable, performant, and maintainable. Frontend engineers should implement various testing methods, such as:
- Unit Testing: Testing individual components with libraries like Jest or Mocha ensures that each part of the application functions correctly in isolation.
- Integration Testing: Ensures that components work together as expected, using tools like React Testing Library or Enzyme.
- End-to-End (E2E) Testing: Simulates user interactions across the application to verify that the entire system behaves as expected, using tools like Cypress or Playwright.
By establishing a robust testing strategy, frontend teams can deploy confidently, knowing that each component of the application is thoroughly vetted.
For frontend engineers, system design is more than just aesthetics; it’s about building applications that are efficient, resilient, and scalable. By mastering component architecture, state management, performance optimization, API integration, accessibility, and testing, frontend engineers can design systems that drive business value, enhance user experience, and scale with evolving demands. Investing in these design principles empowers frontend teams to deliver top-tier applications that not only meet user expectations but set a new standard for quality and innovation.