Testing User Interfaces in Modern JavaScript Frameworks: A Guide for Senior Engineers
As the complexity of web applications continues to increase, especially with the rise of modern JavaScript frameworks like React, Vue, and Angular, the importance of thoroughly testing User Interfaces (UI) has never been greater. Ensuring that a UI behaves as expected under various conditions is key to maintaining the reliability, usability, and quality of your software.
For senior engineers, the challenges of testing UIs in these frameworks go beyond just ensuring buttons click and forms submit correctly—it’s about ensuring the whole experience is stable, performant, and scalable. This article provides an in-depth look at best practices, tools, and techniques for testing UIs in modern JavaScript frameworks, with an emphasis on practical strategies that will help your team ship better code, faster.
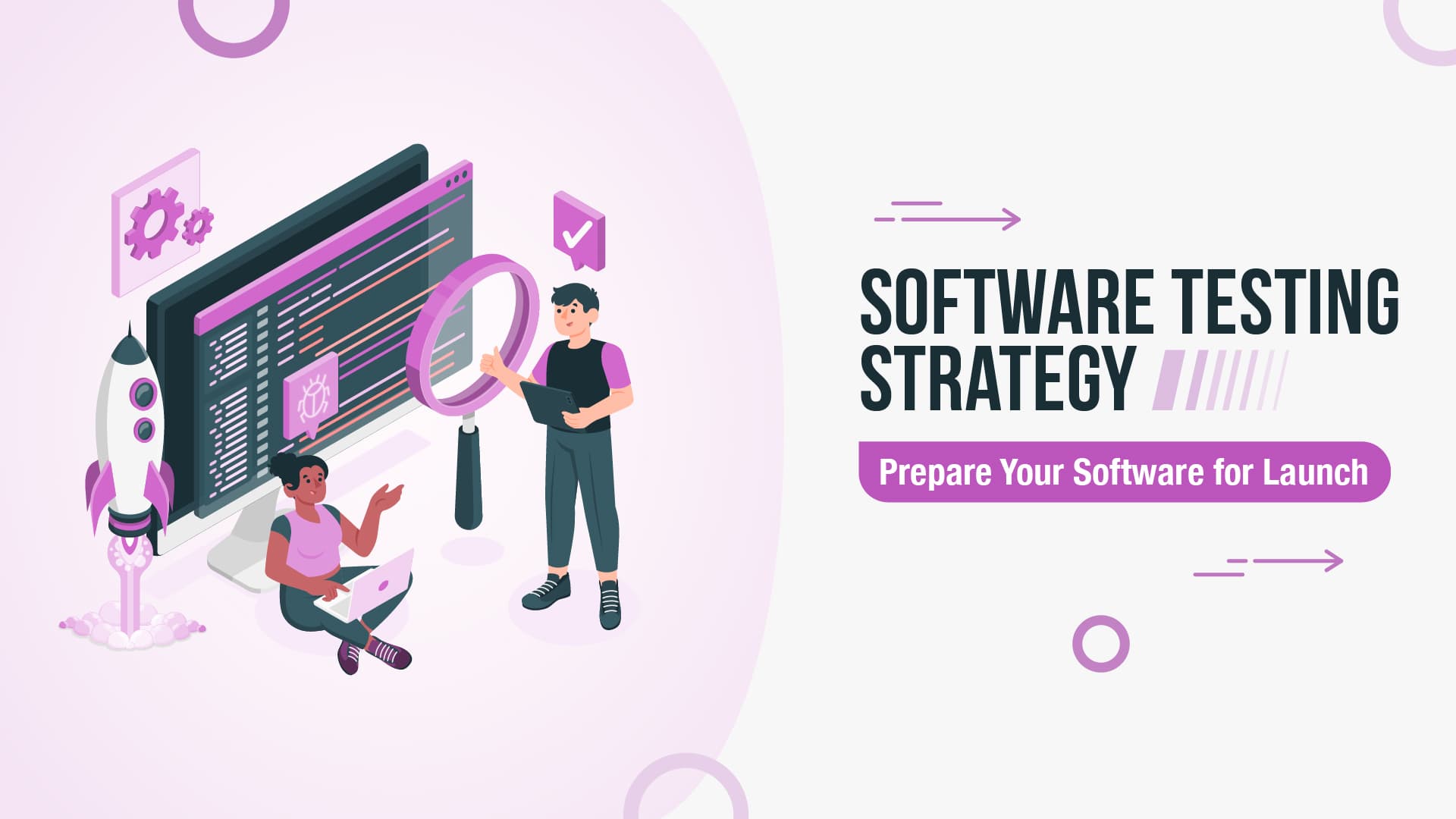
Why UI Testing Matters
User interfaces are the most direct point of interaction between users and your application, which means that a bug or inconsistency can result in a poor user experience, lost conversions, or even decreased brand trust. While backend errors are usually hidden, UI issues are highly visible and can impact the usability and accessibility of your product.
Additionally, modern JavaScript frameworks enable highly dynamic UIs with real-time state changes, reactivity, and complex data flows. As applications grow in size and complexity, so does the challenge of ensuring the UI behaves correctly under different scenarios. This is why automated UI testing, which focuses on ensuring that visual components, interactions, and state updates perform as intended, is an essential part of any modern development pipeline.
Types of UI Testing
UI testing is typically broken into three main categories: unit testing, integration testing, and end-to-end testing. Each serves a different purpose and offers unique advantages when testing the interface of a modern JavaScript application.
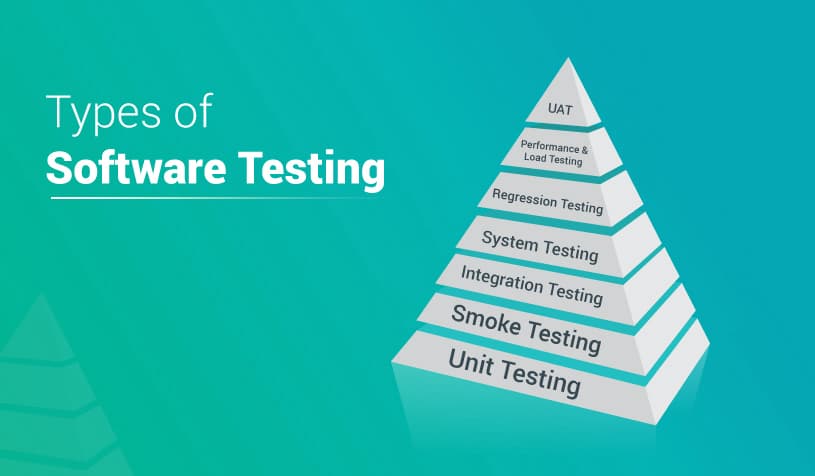
1. Unit Testing
Unit tests verify the smallest components of an application in isolation—typically individual functions or components. In modern JavaScript frameworks like React or Vue, unit testing focuses on components' behavior, ensuring they render correctly and handle props and state changes appropriately.
For unit testing in JavaScript frameworks, libraries like Jest and Mocha are often paired with React Testing Library or Vue Test Utils. These tools allow you to assert the expected behavior of components without needing to rely on the browser environment. This makes it easy to test logic, inputs, and outputs for simple components, but it won’t test how components behave together in a full UI.
Example of a React component unit test with React Testing Library:
import { render, screen, fireEvent } from '@testing-library/react';
import Button from './Button';
test('Button renders correctly and handles click events', () => {
const handleClick = jest.fn();
render(<Button onClick={handleClick}>Click Me</Button>);
fireEvent.click(screen.getByText(/Click Me/i));
expect(handleClick).toHaveBeenCalledTimes(1);
});
2. Integration Testing
While unit tests focus on individual components, integration tests focus on how multiple components work together. Integration tests ensure that data flows correctly between components, state management behaves as expected, and interactions with external dependencies (e.g., APIs) happen correctly.
Integration testing often relies on libraries such as Cypress, Jest, or Enzyme in React, allowing developers to simulate real-world scenarios like user interactions across different components. Integration tests provide confidence that different parts of the UI work well together, but they require more setup than unit tests.
Example of an integration test in React:
import { render, screen } from '@testing-library/react';
import UserProfile from './UserProfile';
test('displays user details fetched from API', async () => {
const mockUser = { name: 'John Doe', email: 'john@example.com' };
global.fetch = jest.fn(() =>
Promise.resolve({
json: () => Promise.resolve(mockUser),
})
);
render(<UserProfile />);
expect(await screen.findByText(/John Doe/)).toBeInTheDocument();
expect(await screen.findByText(/john@example.com/)).toBeInTheDocument();
});
3. End-to-End Testing (E2E)
End-to-end tests simulate real user interactions with the entire application, testing how components, services, and the backend work together from the user's perspective. E2E tests are usually the most comprehensive form of UI testing, covering things like form submissions, navigation, and full user flows.
In modern JavaScript applications, tools like Cypress, Puppeteer, and Playwright are commonly used to handle E2E testing. These tools allow you to simulate real-world conditions, such as network delays, and verify that the application works as expected from the front end down to the backend.
E2E tests, while powerful, tend to be slower and more resource-intensive than unit or integration tests. However, they are crucial for ensuring that the entire system works as expected in real-world usage.
Example of an E2E test using Cypress:
describe('User login flow', () => {
it('logs in a user and redirects to the dashboard', () => {
cy.visit('/login');
cy.get('input[name="email"]').type('user@example.com');
cy.get('input[name="password"]').type('password123');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/dashboard');
cy.get('.welcome-message').should('contain', 'Welcome, user@example.com');
});
});
Best Practices for UI Testing in Modern JavaScript Frameworks
1. Test What Matters
Testing everything in your application is unrealistic and inefficient. Focus on the critical paths—the features and components that users interact with the most. Prioritize testing components that affect core functionality, user experience, and business logic. Testing for edge cases, such as invalid form submissions or error handling, also ensures robustness.
2. Maintain a Testing Pyramid
Following the testing pyramid concept can ensure a balanced testing strategy. The pyramid suggests having more unit tests (which are fast and cover small parts of the application), fewer integration tests, and the least number of E2E tests (which are more expensive to run but offer the highest confidence).
- Unit Tests (bottom of the pyramid): Fast and isolated.
- Integration Tests (middle): Test how components work together.
- E2E Tests (top): Full-stack tests, slow but essential for user journeys.
3. Mock External Dependencies
When writing unit or integration tests, mock any external dependencies (e.g., API calls, third-party services) to avoid network variability and reduce test complexity. Tools like Mock Service Worker (MSW) allow you to intercept network requests and provide mock responses in your test environment.
4. Automate and Integrate Testing with CI/CD
Incorporating testing into your CI/CD pipeline ensures that tests are automatically executed on every push or pull request. Tools like GitHub Actions, CircleCI, and Jenkins can be configured to run tests for your application, ensuring that new changes do not break existing functionality.
Running tests on every change also enforces discipline within the development team and prevents bugs from being introduced into production.
5. Test Accessibility
Modern frameworks make it easy to create highly interactive UIs, but accessibility (a11y) should not be overlooked. Testing for accessibility ensures that your application is usable by everyone, including users with disabilities. Tools like Axe or Testing Library’s a11y integration can help automate accessibility checks within your existing UI tests.
Challenges in UI Testing and How to Overcome Them
1. Flaky Tests
One of the most frustrating issues with UI testing, particularly E2E, is flaky tests—tests that fail intermittently without any real issues in the code. This is often due to timing issues, where tests run too fast or too slow for the application. To combat this, ensure proper use of wait conditions, assertions, and timeouts where necessary. Libraries like Cypress offer robust retry mechanisms that help mitigate flakiness.
2. Test Maintenance
As your application evolves, your tests need to evolve too. Refactoring the application might break several tests, especially if your tests are tightly coupled to implementation details. To minimize maintenance, write tests that focus on outcomes and user behavior, rather than specific implementation details.
3. Performance Bottlenecks
As test suites grow, running all tests can become slow. To speed things up, consider running tests in parallel or breaking them into smaller suites that can be run independently. Tools like Jest’s parallel test runner or Cypress Dashboard can help distribute tests across multiple processes.
Conclusion
For senior engineers, testing user interfaces in modern JavaScript frameworks is about ensuring quality at every level—component, integration, and the entire user journey. With a balanced testing strategy, the right tools, and best practices, you can build robust, maintainable UIs that provide seamless experiences for users while ensuring rapid development cycles. As applications continue to grow in complexity, mastering UI testing is key to delivering scalable, performant, and reliable software.