Achieving Excellence: What to Test in React for Flawless User Experiences.
As software engineering continues to evolve, the complexity of applications grows alongside the demands for reliability, scalability, and flawless user experiences. In the context of modern JavaScript frameworks like React, professional quality assurance (QA) is no longer an afterthought but a foundational component of the development process. With React's component-driven architecture, single-page applications (SPAs), and its heavy use of asynchronous operations, ensuring thorough testing is critical to building high-quality applications.
But what should really be tested in React applications? Effective QA in React must cover several key areas, from UI rendering and component behavior to state management and asynchronous logic. This article explores the essential aspects of React applications that should be tested and how to ensure your testing strategy leads to robust, scalable applications.
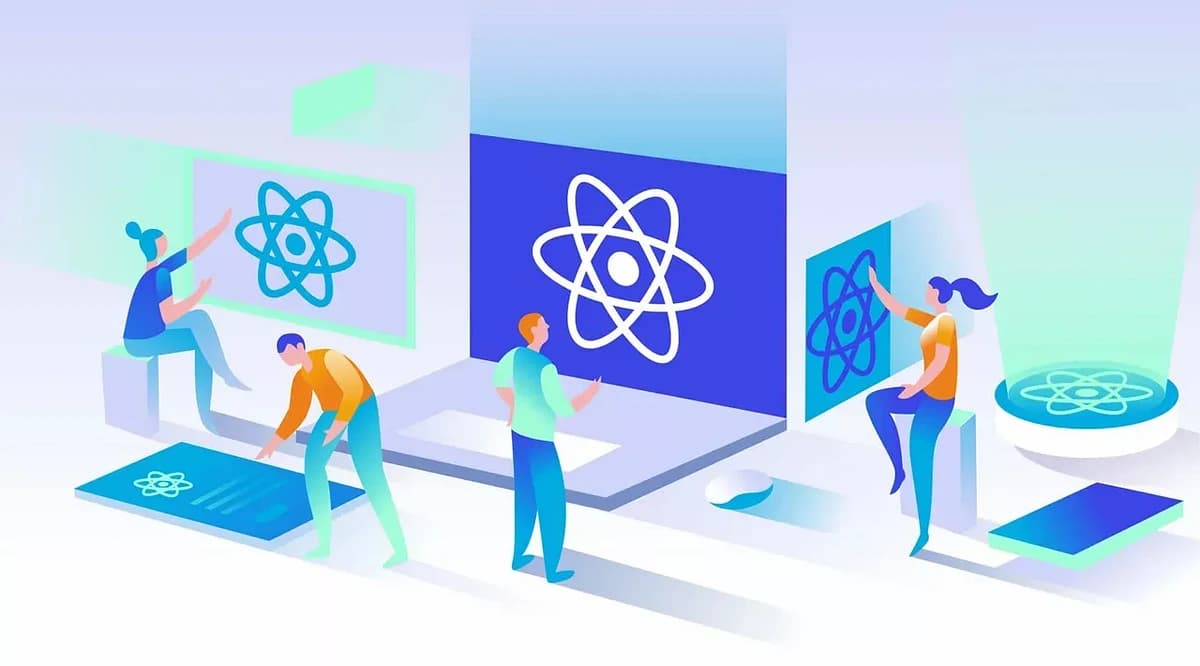
1. Component Rendering and Behavior
React is built around reusable components, making it critical to test that components render correctly under various conditions. Each React component serves as a self-contained unit of the UI, and its appearance and behavior can change based on the props and state it receives.
What to Test:
- Snapshot Testing: Capture a snapshot of the rendered component and compare it to future snapshots to detect unintended UI changes.
- Conditional Rendering: Ensure components render correctly based on different props and state values. Test cases should cover all possible combinations of inputs to verify how the component behaves in each scenario.
- Edge cases: Test how components handle edge cases, such as null or undefined props, empty data, or unusual user inputs.
Snapshot testing tools like Jest provide an easy way to automate these checks, ensuring components don't introduce breaking visual changes over time.
Example:
import { render } from '@testing-library/react';
import renderer from 'react-test-renderer';
import MyComponent from './MyComponent';
it('renders correctly with given props', () => {
const tree = renderer.create(<MyComponent prop1="value" />).toJSON();
expect(tree).toMatchSnapshot();
});
2. State Management and Data Flow
State management is central to React applications, whether you're using React's built-in state (e.g., useState
, useReducer
) or external libraries like Redux or MobX. Testing how data flows through components, how the application state changes, and how side effects are managed is crucial for building maintainable applications.
What to Test:
- State Changes: Verify that components update their state correctly when user interactions or asynchronous operations occur.
- Redux Action and Reducers: If using Redux, unit test your reducers to ensure they return the correct state based on dispatched actions. Similarly, test actions to ensure they trigger the correct state updates.
- Hooks: Ensure that React hooks like
useEffect
,useState
, and custom hooks behave as expected under different conditions. This is especially important for hooks that manage asynchronous logic, such as data fetching or subscriptions.
Example:
import { render, screen } from '@testing-library/react';
import userEvent from '@testing-library/user-event';
import MyComponent from './MyComponent';
it('updates state when button is clicked', async () => {
render(<MyComponent />);
// Simulate a user click event
const button = screen.getByText('Click Me');
await userEvent.click(button);
// Check if the text updated correctly
expect(screen.getByText('Clicked!')).toBeInTheDocument();
});
3. Asynchronous Logic and API Calls
React applications frequently interact with external APIs, making testing asynchronous logic a critical part of QA. Whether you're fetching data or submitting forms, you need to verify that your components handle asynchronous behavior correctly, including error handling, loading states, and successful responses.
What to Test:
- Data Fetching and API Calls: Mock external API calls and test how your components handle different responses, such as successful data fetching, error responses, and network failures.
- Loading States: Test that your UI shows the appropriate loading state while data is being fetched, and that the loading state disappears once the data is available.
- Error Handling: Ensure that your components gracefully handle API failures and show relevant error messages to users.
Mocking libraries like Jest or React Testing Library can be used to mock API requests, simulate network conditions, and control responses.
Example:
import { render, screen } from '@testing-library/react';
import userEvent from '@testing-library/user-event';
import fetchMock from 'jest-fetch-mock';
import MyComponent from './MyComponent';
beforeEach(() => {
fetchMock.resetMocks();
});
it('displays data after fetching', async () => {
// Mock API response
fetchMock.mockResponseOnce(JSON.stringify({ data: 'test data' }));
render(<MyComponent />);
// Simulate a user triggering data fetch
await userEvent.click(screen.getByText('Fetch Data'));
// Wait for the element to appear with the fetched data
expect(await screen.findByText('test data')).toBeInTheDocument();
});
4. User interactions
A critical part of QA in modern React applications involves simulating and testing real-world user interactions. Testing how your components respond to clicks, form submissions, keyboard navigation, and other user events ensures that your UI behaves as intended.
What to Test:
- Form Validations: Test how your form components validate user input, prevent invalid submissions, and display error messages when needed.
- Event Handling: Ensure that event handlers (e.g., button clicks, form submissions) trigger the expected state changes or actions.
- Accessibility (A11y) Compliance: Test that your components are accessible, including keyboard navigation, focus management, and screen reader support.
React Testing Library offers utilities to simulate user interactions, ensuring your components behave properly in response to user events.
Example:
import { render, screen } from '@testing-library/react';
import userEvent from '@testing-library/user-event';
import MyForm from './MyForm';
it('submits form and shows success message', async () => {
render(<MyForm />);
// Simulate user typing into an input field
await userEvent.type(screen.getByLabelText(/name/i), 'John');
// Simulate form submission
await userEvent.click(screen.getByText('Submit'));
// Check if success message is displayed
expect(screen.getByText('Form submitted successfully!')).toBeInTheDocument();
});
5. Performance and Load Testing
As modern web applications grow more complex, performance can become a significant issue, especially for single-page applications like those built with React. Testing the performance of your components and application as a whole ensures that it performs well under heavy load.
What to Test:
- Component Render Performance: Ensure that components render efficiently and do not introduce unnecessary re-renders. React's
useMemo
anduseCallback
hooks can help with optimizing render performance, and these should be covered in performance tests. - Memory Leaks: Verify that your application properly cleans up after itself (e.g., unmounting components, canceling subscriptions or timers) to avoid memory leaks.
- Scalability: Test how your application handles a large number of concurrent users or heavy API traffic. Tools like Lighthouse and React Profiler can be used to measure load times and optimize for better performance.
6. End-to-End Testing (E2E)
While unit and integration tests focus on testing individual components and data flows, end-to-end (E2E) testing validates the entire application flow, from user interaction to API integration and data display. E2E tests simulate real-world user scenarios, ensuring that all pieces of the application work together correctly.
What to Test:
- Critical user Flows: Test end-to-end flows such as logging in, completing forms, checking out, and viewing dashboards. These tests ensure that all layers of the application—from the UI to the backend—work seamlessly.
- Cross-Browser Compatibility: Ensure that your application behaves consistently across different browsers and devices.
- Mobile Responsiveness: If your application supports mobile devices, ensure that key features work properly on smaller screens and touch interfaces.
Tools like Cypress and Selenium are commonly used for automated E2E testing.
Conclusion
In modern JavaScript frameworks like React, professional quality assurance goes beyond basic testing. It requires a comprehensive strategy that covers component rendering, state management, asynchronous logic, and user interactions, all while keeping an eye on performance and scalability. By incorporating unit tests, integration tests, and end-to-end testing, you can ensure that your React application is not only functional but also reliable, maintainable, and scalable.
Ultimately, thorough QA helps you deliver a high-quality product that meets both user expectations and business requirements, while giving your engineering team the confidence to iterate, refactor, and scale the application over time.