Why TypeScript Alone Isn’t Enough: The Critical Role of Unit Testing
As software engineers, we constantly seek ways to improve code reliability and maintainability while reducing the chances of errors slipping into production. One tool that has become popular among developers is TypeScript, which introduces static typing to JavaScript. It catches many common bugs during the development phase by enforcing type checks and providing better IDE support.
Given the robustness of TypeScript, some may wonder if writing unit tests is still necessary. After all, TypeScript already helps prevent many types of errors, right? However, while TypeScript is an incredibly valuable tool, it does not replace the need for unit testing. In fact, TypeScript and unit tests complement each other, ensuring that both the structure and the behavior of your code are sound.
Here are several reasons why you should continue to write unit tests even when using TypeScript:
1. Type Safety Does Not Cover All Logic
TypeScript's primary advantage is that it enforces type safety, ensuring that variables, functions, and objects adhere to expected types. It can prevent issues such as passing a string to a function that expects a number, or trying to access properties that don’t exist on an object. However, business logic errors can still occur even if the types are correct.
For instance, consider a function that calculates a discount based on a customer’s loyalty points. TypeScript ensures that the input and output types are correct, but it won’t detect logical errors in the calculation itself:
function calculateDiscount(points: number): number {
// Incorrect logic, even though the types are correct
if (points > 100) {
return points * 0.1; // This should probably be a fixed discount, not a proportional one
}
return 0;
}
Without unit tests, TypeScript can’t verify whether this discount calculation behaves as expected. Unit tests validate that the logic inside your functions works correctly, regardless of whether the types are correct. Testing ensures that the business rules implemented in the code meet the required specifications.
2. Guarding Against Edge Cases
TypeScript helps you define expected input types, but it doesn’t automatically handle unexpected edge cases or test how your code behaves under different conditions. Let’s say you’re working with a function that accepts an array and processes it. TypeScript will make sure the input is an array, but it won’t check what happens when the array is empty, contains duplicates, or is excessively large.
For example:
function sumElements(arr: number[]): number {
return arr.reduce((acc, curr) => acc + curr, 0);
}
Here, TypeScript ensures that arr
is an array of numbers. However, what happens if the array is empty or contains NaN
? These are potential edge cases that only unit tests can catch. By writing unit tests, you can test various edge cases, such as:
- Empty arrays
- Arrays containing negative numbers
- Large datasets
Unit testing guarantees that your code handles all edge cases appropriately, ensuring robust application behavior in real-world scenarios.
3. Refactoring Confidence
One of the most valuable benefits of unit testing is the confidence it provides when refactoring code. Even with TypeScript, when you make significant changes to your codebase—whether optimizing performance or modifying the business logic—there is a risk of unintentionally breaking existing functionality.
Unit tests act as a safety net during refactoring. They allow you to confidently modify your code, knowing that if a test fails, you’ve caught a regression early. TypeScript will ensure that your types are correct after a refactor, but only unit tests can verify that the behavior of your code hasn’t changed.
For example, refactoring a function’s implementation:
function add(a: number, b: number): number {
return a + b;
}
// After refactoring to handle additional cases
function add(a: number, b: number): number {
if (a === 0) return b;
if (b === 0) return a;
return a + b;
}
Although TypeScript confirms that the types still match the expected inputs and outputs, only unit tests will check if the refactored function behaves correctly under all scenarios.
4. Testing Asynchronous and Side Effects
TypeScript ensures type correctness, but it doesn’t handle asynchronous code or side effects like database interactions, API calls, or file system operations. Modern JavaScript frameworks often rely heavily on promises, async/await patterns, and external data fetching, all of which need to be verified.
Consider this code snippet that fetches data from an API:
async function getUserData(userId: number): Promise<User> {
const response = await fetch(`/api/users/${userId}`);
return await response.json();
}
TypeScript guarantees that userId
is a number and getUserData
returns a Promise<User>
, but it doesn’t validate:
- What happens if the API request fails?
- Does the function handle network timeouts?
- Are there retries in place for failed requests?
Unit tests allow you to mock API calls and simulate various outcomes (successful requests, failures, etc.) to ensure your function behaves correctly under all conditions.
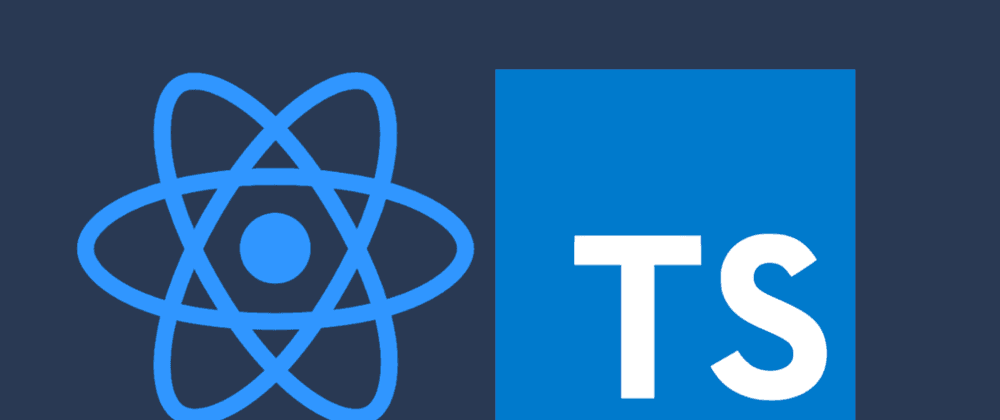
5. Ensuring Code Quality and Maintainability
Writing unit tests improves the overall quality and maintainability of your code. Well-tested codebases are easier to maintain because the tests serve as living documentation of how the system should behave. As the team grows or new engineers join the project, unit tests provide a clear guide for understanding how the code works and what behavior is expected.
TypeScript’s type system can improve the quality of your code by catching many bugs at compile-time, but it doesn't document intent or ensure that the software functions correctly. Unit tests act as executable documentation, ensuring that future developers (or even yourself) know how each component is supposed to behave and why.
6. Proving Code Works in Isolation
Unit tests verify that a piece of code works independently of other components. TypeScript may validate types across your entire codebase, but it doesn’t test isolated units of functionality. Unit tests are vital for breaking down your application into smaller, testable parts, ensuring each unit functions correctly on its own.
For example, a function within a React component might depend on specific props or state. While TypeScript ensures that the correct types are passed into the component, unit tests ensure that each internal function behaves as expected, regardless of the surrounding application.
Conclusion
While TypeScript significantly improves code reliability by enforcing type safety and preventing certain classes of errors, it is not a replacement for unit testing. Unit tests ensure that your code behaves as expected in real-world scenarios, handling edge cases, asynchronous operations, and complex business logic that TypeScript alone cannot verify.
By combining the strengths of TypeScript and unit tests, you create a more robust and resilient codebase, one that can confidently evolve over time without sacrificing quality. TypeScript catches type-related errors at compile time, while unit tests catch logic-related bugs and ensure your code functions correctly in every scenario. Both tools, working together, are essential for building scalable, maintainable, and reliable software.